Django REST Authentication
1. CORS
1.1. Same Origin Policy
The same-origin policy is a critical security mechanism by browsers that restricts how a document or script loaded by one origin can interact with a resource from another origin.
1.2. Definition of Origin
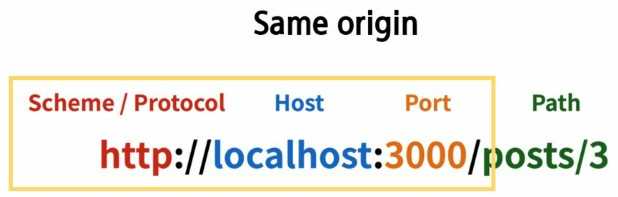
1.3. CORS
Cross-Origin Resource Sharing (CORS) is an HTTP-header based mechanism that allows a server to indicate any origins (domain, scheme, or port) other than its own from which a browser should permit loading resources.
The server determines which origins are accessible to its resources.
1.3.1. django-cors-headers
Library to add CORS header to response
-
Procedure
-
pip install django-cors-headers
-
Add to
INSTALLED_APPS
andMIDDLEWARE
INSTALLED_APPS = [ ..., "corsheaders", ..., ] MIDDLEWARE = [ ..., "corsheaders.middleware.CorsMiddleware", "django.middleware.common.CommonMiddleware", ..., ]
-
Register the accessible domains
CORS_ALLOWED_ORIGINS = [ "https://example.com", ... ] # CORS_ALLOW_ALL_ORIGINS = True
-
2. Authentication
django REST framework Authentication
2.1. Authentication Class
REST framework will attempt to authenticate with each class in the list.
REST_FRAMEWORK = {
# Authentication
'DEFAULT_AUTHENTICATION_CLASSES': [
# ID and Password
'rest_framework.authentication.BasicAuthentication',
# Session
'rest_framework.authentication.SessionAuthentication',
# Token
'rest_framework.authentication.TokenAuthentication',
],
}
2.2. Permission Class
Permissions determine whether a request should be granted or denied access. Permissions in REST framework are always defined as a list of permission classes.
REST_FRAMEWORK = {
# Permission
'DEFAULT_PERMISSION_CLASSES': [
# 'rest_framework.permissions.IsAuthenticated',
'rest_framework.permissions.AllowAny', # 모든 요청에 대해 허용 후, url 개별적으로 제한
],
}
@permission_classes([IsAuthenticated])
def example_view(request, format=None):
content = {
'user': str(request.user), # `django.contrib.auth.User` instance.
'auth': str(request.auth), # None
}
return Response(content)
3. dj-rest-auth
dj-rest-auth doc The library that provides REST API end points related to authentication
3.1. Installation
- User should be customized before start.
AUTH_USER_MODEL = 'accounts.User'
pip install dj-rest-auth
- Add dj_rest_auth app to INSTALLED_APPS in your django settings
INSTALLED_APPS = ( ..., 'rest_framework', 'rest_framework.authtoken', ..., 'dj_rest_auth' )
- Add dj_rest_auth urls
urlpatterns = [ ..., path('dj-rest-auth/', include('dj_rest_auth.urls')) ]
python manage.py migrate
3.2. Registration
Additional packages must be installed to add a membership registration function.
-
pip install 'dj-rest-auth[with_social]'
-
Add apps to INSTALLED_APPS in your django settings
INSTALLED_APPS = [ ..., 'django.contrib.sites', 'allauth', 'allauth.account', 'allauth.socialaccount', 'dj_rest_auth.registration', ]
-
Add SITE_ID = 1 to your django settings
SITE_ID = 1
-
Add dj_rest_auth.registration urls
urlpatterns = [ ..., path('dj-rest-auth/', include('dj_rest_auth.urls')), path('dj-rest-auth/registration/', include('dj_rest_auth.registration.urls')) ]
-
python manage.py migrate